This tutorial shows how to automate multiple devices on the same network by using AskUI. After following this tutorial, you will be able to automate more than one device across different platforms, whether Linux, macOS, Windows or Android, with a single setup with askui library.
In fact, there are many automation tools in the wild that you can use for testing and automating different devices, although most of them require different configurations and different code for different platforms. By using AskUI library, an automation tool that operates on the OS level, you can use the same code for any application running on different devices even without much change. And this makes AskUI a powerful Cross-platform automation tool.
Let's have a look at AskUI and see how we can accomplish a Cross-platform/-device automation test🔥
📌 The following tutorial assumes that you have already installed and set up the AskUI on your local device. See the Getting started for more details:
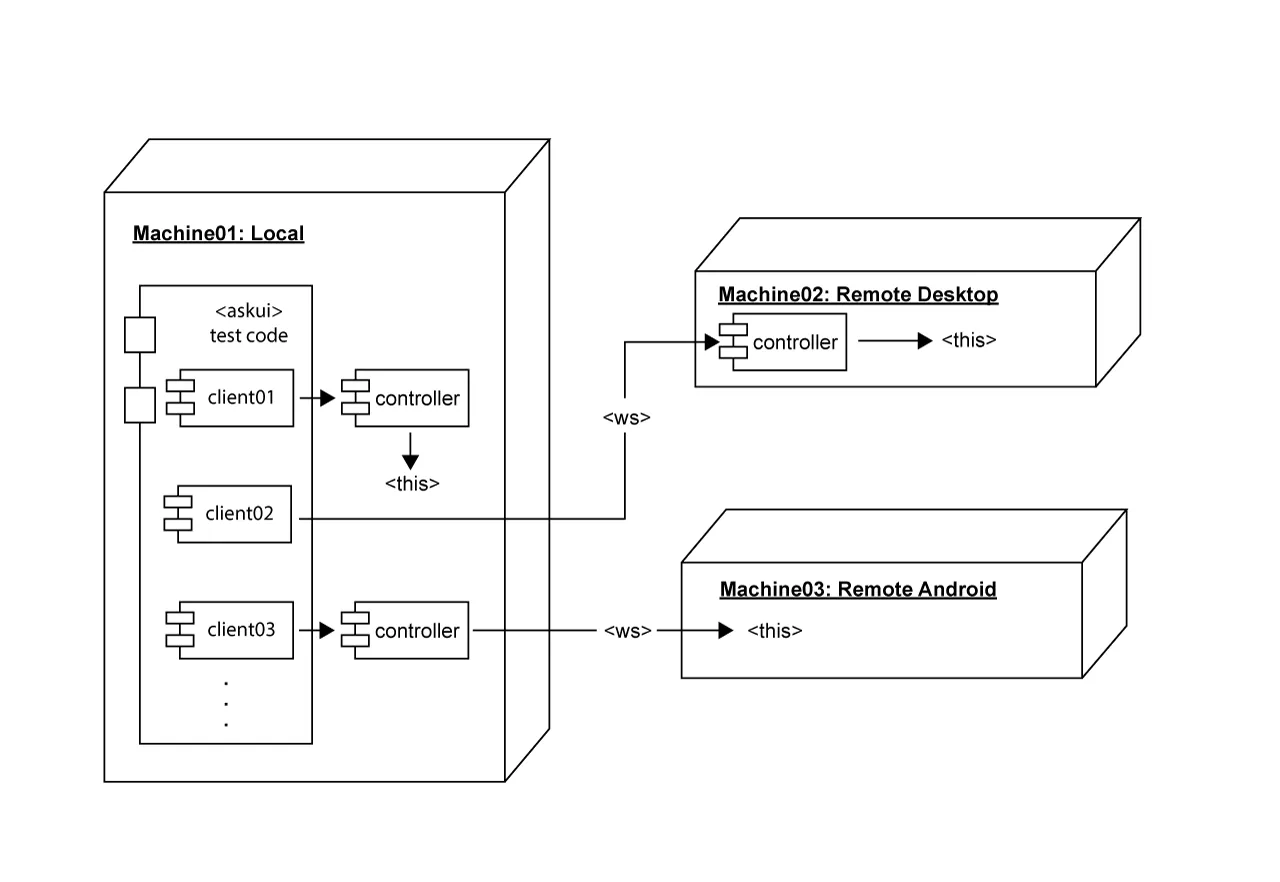
1. Start the AskUI Controller for Each Device
Local Device
Run the following command to start the AskUI Controller for your local device on Port 6769:
# DisplayNum specificies the display the AskUI Controller is controlling
# If you have more than 1 display you have to change accordingly (0 main display)
# The AskUI Controller renders a white border overlay around the controlled display
AskUI-StartController -DisplayNum 0 -RunInBackground -Port 6769
Android Device
Android devices are controlled over the Android Debug Bridge (ADB) by the AskUI Controller running on your local device (desktop).
If you don't have the ADB installed on your local device, set it up by following this tutorial.
Be sure that your Android device is discoverable by the ADB from your local device:
# Make sure that the `USB Debugging Mode` is enabled in the Android device.
# Connect the Android device with a USB cable, and run this command:#
# will print the "device-id"
adb devices
adb -s "device-id" tcpip 9000 # replace "device-id" with your device-id
adb -s "device-id" connect "local-ip-address":9000 # replace the "local-ip-address"
# Run this command, if you want to check the local ip address of the android device
adb -s "device-id" shell ip -f inet addr show wlan0
# Now you can disconnect the USB cable from the Android device.
# Confirm that the Android device is recognised by adb wirelessly.
adb devices
# Start th AskUI Controller
# Substitute <your-device-id> with
# the returned device id
AskUI-StartController -Runtime android -RunInBackground -Port 7001 -DeviceId "<your-device-id>"
Remote Device: Windows, macOS, Linux
For a remote device please use the Silent Installer option of the installer linked in the Getting Started above. After the installation you have to first start the AskUI controller and then to find out the IP of the remote device. You need it for connecting to the AskUI Controller from your local device:
# Start the AskUI Controller on port 7001
AskUI-StartController -DisplayNum 0 -RunInBackground -Port 7001
# Windows only
ipconfig /all
# macOS only
ipconfig getifaddr en0
# Linux only
hostname -I
2. Configure the helpers/askui-helper.ts
For Connecting Remote Devices: Windows, macOS, Linux
Figure out the local ip address of the remote device with the following command:
# Windows only
ipconfig /all
# macOS only
ipconfig getifaddr en0
# Linux only
hostname -I
Change the IP address <local-ip-address> in the askui_example/helpers/askui-helper.ts:
import { UiControlClient} from 'askui';
let localDevice: UiControlClient;
let remoteDevice: UiControlClient;
jest.setTimeout(60 * 1000 * 60);
beforeAll(async () => {
// This client will communicate with
// the controller running on this local device.
localDevice = await UiControlClient.build({
uiControllerUrl: "ws://127.0.0.1:6769",
});
await localDevice.connect();
// This client will communicate with
// the controller running on the remote device.
// Replace the <local-ip-address>
// In case of Android device, replace it with 127.0.0.1
remoteDevice = await UiControlClient.build({
uiControllerUrl: "ws://<remote-ip-address>:7001",
});
await remoteDevice.connect();
});
afterAll(async () => {
localDevice.disconnect();
remoteDevice.disconnect();
});
export { localDevice, remoteDevice };
3. Write the Automation Code
Write the test code in askui_example/my-first-askui-test-suite.test.ts:
import { localDevice, remoteDevice } from './helpers/askui-helper.ts';
describe('jest with askui', () => {
it('should work with multiple devices', async () => {
const everyElement_remote = await remoteDevice.getAll().exec();
console.log(everyElement_remote);
await localDevice.moveMouse(500,500).exec();
const everyElement_local = await localDevice.getAll().exec();
console.log(everyElement_local);
});
});
4. Run the Automation Code
Run the command below to run the AskUI test code:
AskUI-RunProject
5. Conclusion
Now you should be able to automate multiple devices, be it Android, Windows, macOS, Linux or remote devices, in the network.